API DOCS
Unified API (v2.0)
SIMPLE USAGE
This is the easiest way to request a gender determination. Each response is JSON encoded:
GET https://gender-api.com/get?name=Andrea&country=IT&key=<your api key>
Field | Type | Description |
---|---|---|
name | String | Name to query |
key | String | Your API Key |
{"name":"diana","name_sanitized":"Diana","gender":"female","samples":523,"accuracy":93,"duration":"41ms","credits_used":1}
Field | Type | Description |
---|---|---|
name | String | Submitted name in lower case |
name_sanitized | String | The name after we applied our normalizer to it |
gender | String | Possible values: male, female, unknown |
samples | Integer | Number of records found in our database which match your request |
accuracy | Integer | This value determines the reliability of our database. A value of 100 means that the results on your gender request are 100% accurate |
duration | String | Time the server needed to process the request |
credits_used | Integer | The amount of requests used for this query |
LOCALIZATION
Add more accuracy to your request by localizing your query:
GET https://gender-api.com/get?name=Andrea&country=IT&key=<your api key>
GET https://gender-api.com/get?name=Andrea&country=DE&key=<your api key>
Field | Type | Description |
---|---|---|
name | String | Name to query |
country | String | ISO 3166 ALPHA-2 Country Code |
key | String | Your API Key |
//In Italy, Andrea is male.
{"name":"andrea","name_sanitized":"Andrea","country":"IT","gender":"male","samples":1068,"accuracy":95,"duration":"15ms","credits_used":1}
//In Germany, Andrea is female.
{"name":"andrea","name_sanitized":"Andrea","country":"DE","gender":"female","samples":4089,"accuracy":85,"duration":"33ms","credits_used":1}
Field | Type | Description |
---|---|---|
name | String | Submitted name in lower case |
name_sanitized | String | The name after we applied our normalizer to it |
country | String | Submitted country code |
gender | String | Possible values: male, female, unknown |
samples | Integer | Number of records found in our database which match your request |
accuracy | Integer | This value determines the reliability of our database. A value of 100 means that the results on your gender request are 100% accurate |
duration | String | Time the server needed to process the request |
credits_used | Integer | The amount of requests used for this query |
Valid country codes:
LOCALIZATION - COUNTRY BY IP
Choose the country of your customer based on their IP address:
GET https://gender-api.com/get?name=john&ip=54.201.16.177&key=<your api key>
GET https://gender-api.com/get?name=tanja&ip=178.27.177.23&key=<your api key>
Field | Type | Description |
---|---|---|
name | String | Name to query |
ip | String | Valid IP address |
key | String | Your API Key |
{"name":"john","name_sanitized":"John","country":"US","gender":"male","samples":9991,"accuracy":99,"duration":"44ms","credits_used":1}
{"name":"tanja","name_sanitized":"Tanja","country":"DE","gender":"female","samples":143,"accuracy":97,"duration":"508ms","credits_used":1}
Field | Type | Description |
---|---|---|
name | String | Submitted name in lower case |
name_sanitized | String | The name after we applied our normalizer to it |
country | String | Submitted country code |
gender | String | Possible values: male, female, unknown |
samples | Integer | Number of records found in our database which match your request |
accuracy | Integer | This value determines the reliability of our database. A value of 100 means that the results on your gender request are 100% accurate |
duration | String | Time the server needed to process the request |
credits_used | Integer | The amount of requests used for this query |
Gender-API.com includes GeoLite2 data created by MaxMind, available from http://www.maxmind.com.
LOCALIZATION - COUNTRY BY BROWSER LANGUAGE / LOCALE
Choose the country of your customer based on their browser locale:
GET https://gender-api.com/get?name=john&locale=en_US&key=<your api key>
GET https://gender-api.com/get?name=tanja&locale=de_DE&key=<your api key>
Field | Type | Description |
---|---|---|
name | String | Name to query |
locale | String | Browser locale |
key | String | Your API Key |
{"name":"john","name_sanitized":"John","country":"US","gender":"male","samples":991,"accuracy":99,"duration":"47ms","credits_used":1}
{"name":"tanja","name_sanitized":"Tanja","country":"DE","gender":"female","samples":143,"accuracy":97,"duration":"99ms","credits_used":1}
Field | Type | Description |
---|---|---|
name | String | Submitted name in lower case |
name_sanitized | String | The name after we applied our normalizer to it |
country | String | Submitted country code |
gender | String | Possible values: male, female, unknown |
samples | Integer | Number of records found in our database which match your request |
accuracy | Integer | This value determines the reliability of our database. A value of 100 means that the results on your gender request are 100% accurate |
duration | String | Time the server needed to process the request |
credits_used | Integer | The amount of requests used for this query |
QUERY MULTIPLE NAMES
Sometimes it is necessary to fetch multiple names with one query:
GET https://gender-api.com/get?name=lisa;jess;thomas&multi=true&key=<your api key>
GET https://gender-api.com/get?name=anna;jack;stephen&country=DE;US;CA&multi=true&key=<your api key>
Field | Type | Description |
---|---|---|
name | String | Semicolon separated list of names to query |
country | String | Semicolon separated list of country codes. The country codes must be in the same order as the names. If only one country code is provided this code will be used for all names. |
multi | Boolean (true|false) | Tells the API to return the result as an array |
key | String | Your API Key |
{"name":"lisa;jess;thomas","result":[
{"name":"lisa","name_sanitized":"Lisa","country":"","gender":"female","samples":806,"accuracy":98},
{"name":"jess","name_sanitized":"Jess","country":"","gender":"female","samples":28,"accuracy":75},
{"name":"thomas","name_sanitized":"Thomas","country":"","gender":"male","samples":2239,"accuracy":99}],
"country":"","duration":"425ms","credits_used":3}
{"name":"anna;jack;stephen","result":[
{"name":"anna","name_sanitized":"Anna","country":"DE","gender":"female","samples":1245,"accuracy":98},
{"name":"jack","name_sanitized":"Jack","country":"US","gender":"male","samples":667,"accuracy":90},
{"name":"stephen","name_sanitized":"Stephen","country":"CA","gender":"male","samples":77,"accuracy":82}],
"country":"DE;US;CA","duration":"668ms","credits_used":3}
Field | Type | Description |
---|---|---|
name | String | Submitted names in lower case. The maximum number of names is limited to 100. |
result | Array | See response under "Simple Usage" |
country | String | Submitted list of country codes |
duration | String | Time the server needed to process the request |
credits_used | Integer | The amount of requests used for this query |
EMAIL ADDRESS
You can also query a gender using an email address containing a first name:
GET https://gender-api.com/get?email=markus.johnson@gmail.com&key=<your api key>
GET https://gender-api.com/get?email=jack.meyer@gmail.com&country=US&key=<your api key>
Field | Type | Description |
---|---|---|
name | String | Name to query |
String | Email address | |
key | String | Your API Key |
{"email":"markus.johnson@gmail.com","last_name":"Johnson","first_name":"Markus","mail_provider":"gmail","name":"markus","name_sanitized":"Markus","country":"","gender":"male","samples":150,"accuracy":99,"duration":"669ms","credits_used":1}
{"email":"jack.meyer@gmail.com","last_name":"Meyer","first_name":"Jack","mail_provider":"gmail","name":"jack","name_sanitized":"Jack","country":"US","gender":"male","samples":667,"accuracy":90,"duration":"1011ms","credits_used":1}
Field | Type | Description |
---|---|---|
String | The submitted email address | |
last_name | String | The last name found |
first_name | String | The first name found |
mail_provider | String | Name of the email provider |
name | String | First Name found in lowercase |
name_sanitized | String | The name after we applied our normalizer to it |
country | String | Submitted country code |
gender | String | Possible values: male, female, unknown |
samples | Integer | Number of records found in our database which match your request |
accuracy | Integer | This value determines the reliability of our database. A value of 100 means that the results on your gender request are 100% accurate |
duration | String | Time the server needed to process the request |
credits_used | Integer | The amount of requests used for this query |
SPLIT FIRST AND LAST NAME
If you use a combined field for first and last name on your page, use this API to extract the parts:
GET https://gender-api.com/get?split=theresa%20miller&key=<your api key>
GET https://gender-api.com/get?split=tim%20johnson&country=US&key=<your api key>
GET https://gender-api.com/get?split=markus%20stefan%20nonExistingLastName&strict=true&key=<your api key>
Field | Type | Description |
---|---|---|
split | String | Full name |
country | String | ISO 3166 ALPHA-2 Country Code |
strict | Boolean (true|false) | When the strict mode is enabled, we will return null in the last_name field if the last_name cannot be found in our database. When the strict mode is disabled, we will try to extract the last name even if it cannot be found in our database |
key | String | Your API Key |
{"last_name":"Miller","first_name":"Theresa","strict":false,"name":"theresa","name_sanitized":"Theresa","country":"","gender":"female","samples":70,"accuracy":74,"duration":"56ms","credits_used":1}
{"last_name":"Johnson","first_name":"Tim","strict":false,"name":"tim","name_sanitized":"Tim","country":"US","gender":"male","samples":7,"accuracy":100,"duration":"58ms","credits_used":1}
{"last_name":"","first_name":"Markus Stefan","strict":true,"name":"markus","name_sanitized":"Markus","country":"","gender":"male","samples":150,"accuracy":99,"duration":"85ms","credits_used":1}
Field | Type | Description |
---|---|---|
last_name | String | The last name found |
first_name | String | The first name found |
strict | Boolean | True if the strict mode is enabled |
name | String | First Name found in lowercase |
name_sanitized | String | The name after we applied our normalizer to it |
country | String | Submitted country code |
gender | String | Possible values: male, female, unknown |
samples | Integer | Number of records found in our database which match your request |
accuracy | Integer | This value determines the reliability of our database. A value of 100 means that the results on your gender request are 100% accurate |
duration | String | Time the server needed to process the request |
credits_used | Integer | The amount of requests used for this query |
Please indicate your customers enter their names in the form "First Name, Last Name", which makes our response more accurate.
GET STATS
With this call you can pull some stats on your account:
GET https://gender-api.com/get-stats?&key=<your api key>
Field | Type | Description |
---|---|---|
key | String | Your API Key |
{"key":"yourkey","is_limit_reached":false,"remaining_requests":43566,"amount_month_start":90000,"amount_month_bought":100000,"duration":"37ms"}
Field | Type | Description |
---|---|---|
key | String | Your API Key |
is_limit_reached | Boolean | Returns true if there are no more requests left |
remaining_requests | Integer | Counts remaining requests |
amount_month_start | Integer | Requests left at the beginning of the month |
amount_month_bought | Integer | Requests bought this month |
duration | String | Time the server needed to process the request |
Please note:
This API endpoint is still experimental. Which means:
- it may not deliver the expected quality of results in some regions yet
- the syntax may change in the future
- most clients do not support this endpoint yet
Get Country Of Origin
Get a first name's country of origin:
GET https://gender-api.com/get-country-of-origin?name=Markus&key=<your api key>
Field | Type | Description |
---|---|---|
name | String | Name to query |
key | String | Your API Key |
{
"name": "markus",
"country_of_origin": [
{
"countryName": "Finland",
"country": "FI",
"probability": 0.74,
"continentalRegion": "Europe",
"statisticalRegion": "Northern Europe"
},
{
"countryName": "Sweden",
"country": "SE",
"probability": 0.22,
"continentalRegion": "Europe",
"statisticalRegion": "Northern Europe"
},
{
"countryName": "Other",
"country": "--",
"probability": 0.04
}
],
"name_sanitized": "Markus",
"gender": "male",
"samples": 150,
"accuracy": 99,
"country_of_origin_map_url": "https://gender-api.com/en/map/37/3da90b0fe64e5102"
"credits_used": 2,
"duration": "165ms"
}
Field | Type | Description |
---|---|---|
name | String | Submitted name in lower case |
name_sanitized | String | The name after we applied our normalizer to it |
gender | String | Possible values: male, female, unknown |
samples | Integer | Number of records found in our database which match your request |
accuracy | Integer | This value determines the reliability of our database. A value of 100 means that the results on your gender request are 100% accurate |
country_of_origin | Array | Top 25 countries of origin. See "%sComplete List Of Possible Countries%s" for a list of supported countries |
country_of_origin_map_url | String | A link to a map that displays the result in a rendered form. The map can be accessed for 10 minutes. For example you can embed this map in your product by using an iframe. |
duration | String | Time the server needed to process the request |
credits_used | Integer | The amount of requests used for this query |
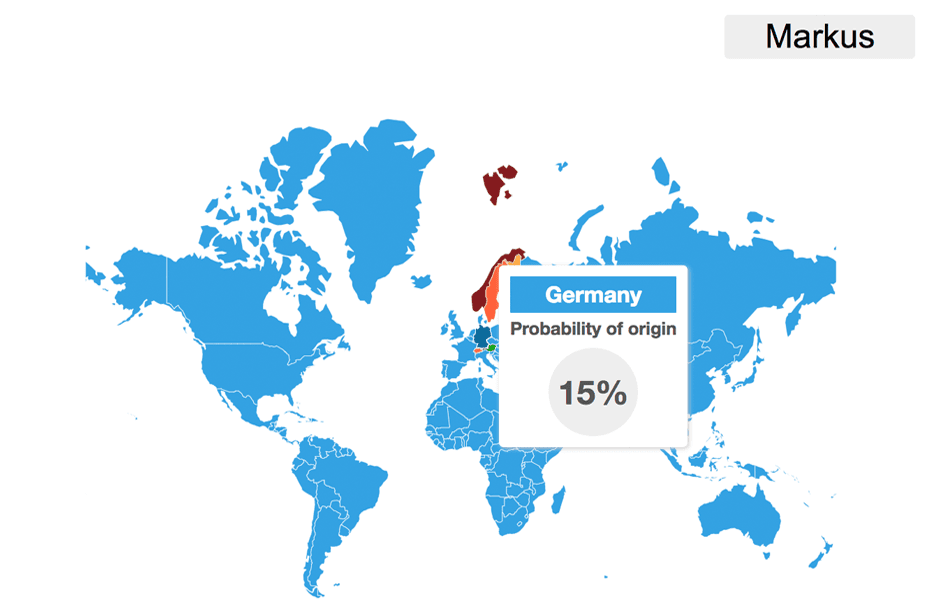
Country Code | Country Name | Statistical Region | Continental Region |
---|---|---|---|
CN | China | Eastern Asia | Asia |
IN | India | Southern Asia | Asia |
US | United States | Northern America | Americas |
ID | Indonesia | South-eastern Asia | Asia |
BR | Brazil | South America | Americas |
PK | Pakistan | Southern Asia | Asia |
NG | Nigeria | Western Africa | Africa |
BD | Bangladesh | Southern Asia | Asia |
RU | Russia | Eastern Europe | Europe |
MX | Mexico | Central America | Americas |
JP | Japan | Eastern Asia | Asia |
ET | Ethiopia | Eastern Africa | Africa |
PH | Philippines | South-eastern Asia | Asia |
EG | Egypt | Northern Africa | Africa |
VN | Vietnam | South-eastern Asia | Asia |
DE | Germany | Western Europe | Europe |
CD | Democratic Republic of the Congo | Middle Africa | Africa |
IR | Iran | Southern Asia | Asia |
TR | Turkey | Western Asia | Asia |
TH | Thailand | South-eastern Asia | Asia |
GB | United Kingdom | Northern Europe | Europe |
FR | France | Western Europe | Europe |
IT | Italy | Southern Europe | Europe |
TZ | Tanzania | Eastern Africa | Africa |
ZA | South Africa | Southern Africa | Africa |
MM | Myanmar | South-eastern Asia | Asia |
KR | South Korea | Eastern Asia | Asia |
CO | Colombia | South America | Americas |
KE | Kenya | Eastern Africa | Africa |
ES | Spain | Southern Europe | Europe |
AR | Argentina | South America | Americas |
UA | Ukraine | Eastern Europe | Europe |
UG | Uganda | Eastern Africa | Africa |
DZ | Algeria | Northern Africa | Africa |
SD | Sudan | Northern Africa | Africa |
IQ | Iraq | Western Asia | Asia |
PL | Poland | Eastern Europe | Europe |
CA | Canada | Northern America | Americas |
MA | Morocco | Northern Africa | Africa |
AF | Afghanistan | Southern Asia | Asia |
SA | Saudi Arabia | Western Asia | Asia |
PE | Peru | South America | Americas |
VE | Venezuela | South America | Americas |
UZ | Uzbekistan | Central Asia | Asia |
MY | Malaysia | South-eastern Asia | Asia |
AO | Angola | Middle Africa | Africa |
MZ | Mozambique | Eastern Africa | Africa |
NP | Nepal | Southern Asia | Asia |
GH | Ghana | Western Africa | Africa |
YE | Yemen | Western Asia | Asia |
MG | Madagascar | Eastern Africa | Africa |
KP | North Korea | Eastern Asia | Asia |
AU | Australia | AustraliaandNew Zealand | Oceania |
CI | Ivory Coast | Western Africa | Africa |
CM | Cameroon | Middle Africa | Africa |
TW | Taiwan | Eastern Asia | Asia |
NE | Niger | Western Africa | Africa |
LK | Sri Lanka | Southern Asia | Asia |
RO | Romania | Eastern Europe | Europe |
BF | Burkina Faso | Western Africa | Africa |
MW | Malawi | Eastern Africa | Africa |
ML | Mali | Western Africa | Africa |
SY | Syria | Western Asia | Asia |
KZ | Kazakhstan | Central Asia | Asia |
CL | Chile | South America | Americas |
ZM | Zambia | Eastern Africa | Africa |
NL | Netherlands | Western Europe | Europe |
GT | Guatemala | Central America | Americas |
EC | Ecuador | South America | Americas |
ZW | Zimbabwe | Eastern Africa | Africa |
KH | Cambodia | South-eastern Asia | Asia |
SN | Senegal | Western Africa | Africa |
TD | Chad | Middle Africa | Africa |
SO | Somalia | Eastern Africa | Africa |
GN | Guinea | Western Africa | Africa |
SD | Sudan | Northern Africa | Africa |
RW | Rwanda | Eastern Africa | Africa |
TN | Tunisia | Northern Africa | Africa |
CU | Cuba | Caribbean | Americas |
BE | Belgium | Western Europe | Europe |
BJ | Benin | Western Africa | Africa |
GR | Greece | Southern Europe | Europe |
BO | Bolivia | South America | Americas |
HT | Haiti | Caribbean | Americas |
BI | Burundi | Eastern Africa | Africa |
DO | Dominican Republic | Caribbean | Americas |
CZ | Czech Republic | Eastern Europe | Europe |
PT | Portugal | Southern Europe | Europe |
SE | Sweden | Northern Europe | Europe |
AZ | Azerbaijan | Western Asia | Asia |
HU | Hungary | Eastern Europe | Europe |
JO | Jordan | Western Asia | Asia |
BY | Belarus | Eastern Europe | Europe |
AE | United Arab Emirates | Western Asia | Asia |
HN | Honduras | Central America | Americas |
TJ | Tajikistan | Central Asia | Asia |
RS | Serbia | Southern Europe | Europe |
AT | Austria | Western Europe | Europe |
CH | Switzerland | Western Europe | Europe |
IL | Israel | Western Asia | Asia |
PG | Papua New Guinea | Melanesia | Oceania |
TG | Togo | Western Africa | Africa |
SL | Sierra Leone | Western Africa | Africa |
HK | Hong Kong | Eastern Asia | Asia |
BG | Bulgaria | Eastern Europe | Europe |
LA | Laos | South-eastern Asia | Asia |
PY | Paraguay | South America | Americas |
SV | El Salvador | Central America | Americas |
LY | Libya | Northern Africa | Africa |
NI | Nicaragua | Central America | Americas |
LB | Lebanon | Western Asia | Asia |
KG | Kyrgyzstan | Central Asia | Asia |
TM | Turkmenistan | Central Asia | Asia |
DK | Denmark | Northern Europe | Europe |
SG | Singapore | South-eastern Asia | Asia |
FI | Finland | Northern Europe | Europe |
SK | Slovakia | Eastern Europe | Europe |
NO | Norway | Northern Europe | Europe |
CG | Congo | Middle Africa | Africa |
ER | Eritrea | Eastern Africa | Africa |
PS | Palestine | Western Asia | Asia |
CR | Costa Rica | Central America | Americas |
IE | Ireland | Northern Europe | Europe |
LR | Liberia | Western Africa | Africa |
NZ | New Zealand | AustraliaandNew Zealand | Oceania |
CF | Central African Republic | Middle Africa | Africa |
OM | Oman | Western Asia | Asia |
MR | Mauritania | Western Africa | Africa |
HR | Croatia | Southern Europe | Europe |
KW | Kuwait | Western Asia | Asia |
PA | Panama | Central America | Americas |
MD | Moldova | Eastern Europe | Europe |
GE | Georgia | Western Asia | Asia |
PR | Puerto Rico | Caribbean | Americas |
BA | Bosnia and Herzegovina | Southern Europe | Europe |
UY | Uruguay | South America | Americas |
MN | Mongolia | Eastern Asia | Asia |
AM | Armenia | Western Asia | Asia |
AL | Albania | Southern Europe | Europe |
JM | Jamaica | Caribbean | Americas |
LT | Lithuania | Northern Europe | Europe |
QA | Qatar | Western Asia | Asia |
NA | Namibia | Southern Africa | Africa |
BW | Botswana | Southern Africa | Africa |
LS | Lesotho | Southern Africa | Africa |
GM | The Gambia | Western Africa | Africa |
MK | Republic of Macedonia | Southern Europe | Europe |
SI | Slovenia | Southern Europe | Europe |
GA | Gabon | Middle Africa | Africa |
LV | Latvia | Northern Europe | Europe |
GW | Guinea-Bissau | Western Africa | Africa |
BH | Bahrain | Western Asia | Asia |
TT | Trinidad and Tobago | Caribbean | Americas |
SZ | Swaziland | Southern Africa | Africa |
EE | Estonia | Northern Europe | Europe |
TL | East Timor | South-eastern Asia | Asia |
GQ | Equatorial Guinea | Middle Africa | Africa |
MU | Mauritius | Eastern Africa | Africa |
CY | Cyprus | Western Asia | Asia |
DJ | Djibouti | Eastern Africa | Africa |
FJ | Fiji | Melanesia | Oceania |
RE | Réunion | Eastern Africa | Africa |
KM | Comoros | Eastern Africa | Africa |
BT | Bhutan | Southern Asia | Asia |
GY | Guyana | South America | Americas |
ME | Montenegro | Southern Europe | Europe |
MO | Macau | Eastern Asia | Asia |
SB | Solomon Islands | Melanesia | Oceania |
LU | Luxembourg | Western Europe | Europe |
SR | Suriname | South America | Americas |
EH | Western Sahara | Northern Africa | Africa |
CV | Cape Verde | Western Africa | Africa |
GP | Guadeloupe | Caribbean | Americas |
MV | Maldives | Southern Asia | Asia |
MT | Malta | Southern Europe | Europe |
BN | Brunei | South-eastern Asia | Asia |
BS | Bahamas | Caribbean | Americas |
MQ | Martinique | Caribbean | Americas |
BZ | Belize | Central America | Americas |
IS | Iceland | Northern Europe | Europe |
BB | Barbados | Caribbean | Americas |
PF | French Polynesia | Polynesia | Oceania |
GF | French Guiana | South America | Americas |
NC | New Caledonia | Melanesia | Oceania |
VU | Vanuatu | Melanesia | Oceania |
YT | Mayotte | Eastern Africa | Africa |
ST | Sao Tome and Principe | Middle Africa | Africa |
WS | Samoa | Polynesia | Oceania |
LC | Saint Lucia | Caribbean | Americas |
JE | Guernsey and Jersey | Northern Europe | Europe |
GU | Guam | Micronesia | Oceania |
CW | Curaçao | Caribbean | Americas |
KI | Kiribati | Micronesia | Oceania |
VC | Saint Vincent and the Grenadines | Caribbean | Americas |
TO | Tonga | Polynesia | Oceania |
GD | Grenada | Caribbean | Americas |
FM | Federated States of Micronesia | Micronesia | Oceania |
AW | Aruba | Caribbean | Americas |
VI | United States Virgin Islands | Caribbean | Americas |
AG | Antigua and Barbuda | Caribbean | Americas |
SC | Seychelles | Eastern Africa | Africa |
IM | Isle of Man | Northern Europe | Europe |
AD | Andorra | Southern Europe | Europe |
DM | Dominica | Caribbean | Americas |
KY | Cayman Islands | Caribbean | Americas |
BM | Bermuda | Northern America | Americas |
GL | Greenland | Northern America | Americas |
AS | American Samoa | Polynesia | Oceania |
KN | Saint Kitts and Nevis | Caribbean | Americas |
MP | Northern Mariana Islands | Micronesia | Oceania |
MH | Marshall Islands | Micronesia | Oceania |
FO | Faroe Islands | Northern Europe | Europe |
SX | Sint Maarten | Caribbean | Americas |
MC | Monaco | Western Europe | Europe |
LI | Liechtenstein | Western Europe | Europe |
TC | Turks and Caicos Islands | Caribbean | Americas |
GI | Gibraltar | Southern Europe | Europe |
SM | San Marino | Southern Europe | Europe |
VG | British Virgin Islands | Caribbean | Americas |
BQ | Caribbean Netherlands | Caribbean | Americas |
PW | Palau | Micronesia | Oceania |
CK | Cook Islands | Polynesia | Oceania |
AI | Anguilla | Caribbean | Americas |
WF | Wallis and Futuna | Polynesia | Oceania |
NR | Nauru | Micronesia | Oceania |
TV | Tuvalu | Polynesia | Oceania |
PM | Saint Pierre and Miquelon | Northern America | Americas |
MS | Montserrat | Caribbean | Americas |
SH | Saint Helena Ascension and Tristan da Cunha | Western Africa | Africa |
FK | Falkland Islands | South America | Americas |
NU | Niue | Polynesia | Oceania |
TK | Tokelau | Polynesia | Oceania |
VA | Vatican City | Southern Europe | Europe |
ERROR CODES
{"errno":30,"errmsg":"limit reached"}
errno (Error Number) | errmsg (Error Message) | Description |
---|---|---|
10 | invalid country code | See "Valid country codes" |
11 | number of country codes not equal to number of names | The name count does not match the count of country codes |
20 | name not set | No name was received by the server |
21 | too many names | Only 100 names allowed at once |
30 | limit reached | Query limit reached |
40 | invalid or missing key | Your server key was not found in our database |
500 | unknown error | Internal server error |